goto' Statement in C language. goto is a jumping statement in c language, which transfer the program's control from one statement to another statement (where label is defined). goto can transfer the program's within the same block and there must a label, where you want to transfer program's control.
A goto statement in C programming provides an unconditional jump from the 'goto' to a labeled statement in the same function.
NOTE − Use of goto statement is highly discouraged in any programming language because it makes difficult to trace the control flow of a program, making the program hard to understand and hard to modify. Any program that uses a goto can be rewritten to avoid them.
Syntax
The syntax for a goto statement in C is as follows −
goto label; .. . label: statement;
Here label can be any plain text except C keyword and it can be set anywhere in the C program above or below to goto statement.
Flow Diagram
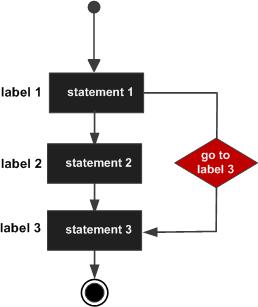
Example:
// Program to calculate the sum and average of positive numbers
// If the user enters a negative number, the sum and average are displayed.
# include <stdio.h>
int main()
{
const int maxInput = 5;
int i;
double number, average, sum=0.0;
for(i=1; i<=maxInput; ++i)
{
printf("%d. Enter a number: ", i);
scanf("%lf",&number);
if(number < 0.0)
goto jump;
sum += number;
}
jump:
average=sum/(i-1);
printf("Sum = %.2f\n", sum);
printf("Average = %.2f", average);
return 0;
}
Output
1. Enter a number: 3 2. Enter a number: 4.3 3. Enter a number: 9.3 4. Enter a number: -2.9 Sum = 16.60
No comments:
Post a Comment